MiniBar specification tutorial
import Specter.Framework
import Bender
context "At Bender's bar":
_bar as duck #our subject is defined in the setup block below
setup:
subject _bar = Bender.MiniBar()
#one-liner shorthand
specify { _bar.DrinkOneBeer() }.Must.Not.Throw()
specify "If I drink 5 beers then I owe 5 bucks":
for i in range(5):
_bar.DrinkOneBeer()
_bar.Balance.Must.Equal(-5)
specify "If I drink more than ten beers then I get drunk":
for i in range(10):
_bar.DrinkOneBeer()
{ _bar.DrinkOneBeer() }.Must.Throw()
1) prepare an empty Bender namespace
Please note though we implement Bender namespace in Boo in this tutorial you can actually use C# or any .NET language of your choice.
Create a file named bender.boo with the following content :
namespace Bender
Compile this into a library with booc -t:library bender.boo
This will produce bender.dll
2) compile the spec
Copy-paste the MiniBarSpec above in a file named MiniBarSpec.boo
Compile it with booc -r:bender.dll MiniBarSpec.boo
This will produce MiniBarSpec.exe
3) run the spec
execute MiniBarSpec.exe, you will get the following result :
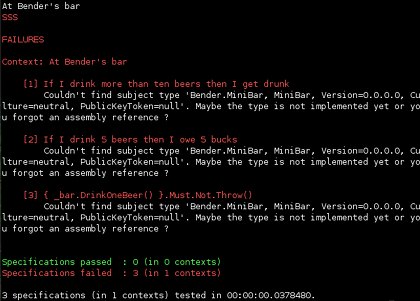
3) add the MiniBar class into Bender namespace
Good! We have SubjectTypeNotFound
errors since we have not implemented the MiniBar class yet.
Let's add the class in bender.boo :
namespace Bender
class MiniBar:
pass
Recompile bender.dll again (booc -t:library bender.boo
), then re-run the spec (re-compiling the spec is not required) : we'll get MissingMethod
errors.
4) add the required method and property in the MiniBar class
Let's add the method and the property in bender.boo :
namespace Bender
class MiniBar:
def DrinkOneBeer():
pass
[getter(Balance)]
_balance = 0
Recompile bender.dll again, then re-run the spec, now that we have all the required members, we'll get this result :
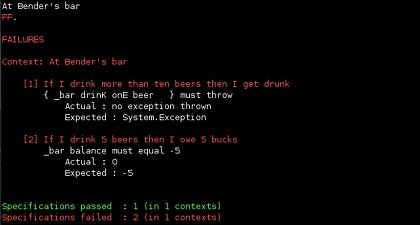
Good! The first specify works the implementation still not matches the other two.
4) implement correctly the DrinkOneBeer
method
Edit bender.boo again and implement method :
namespace Bender
class MiniBar:
def DrinkOneBeer():
_balance--
if _balance < -10:
raise System.Exception("i'm drunk")
[getter(Balance)]
_balance = 0
Recompile bender.dll again, then re-run the spec, now we'll get this result :
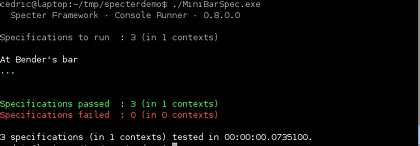
Sweet! We made it!
Want more ?
Look at the other documentation links on the homepage.